BASH Functions – Shell Scripting
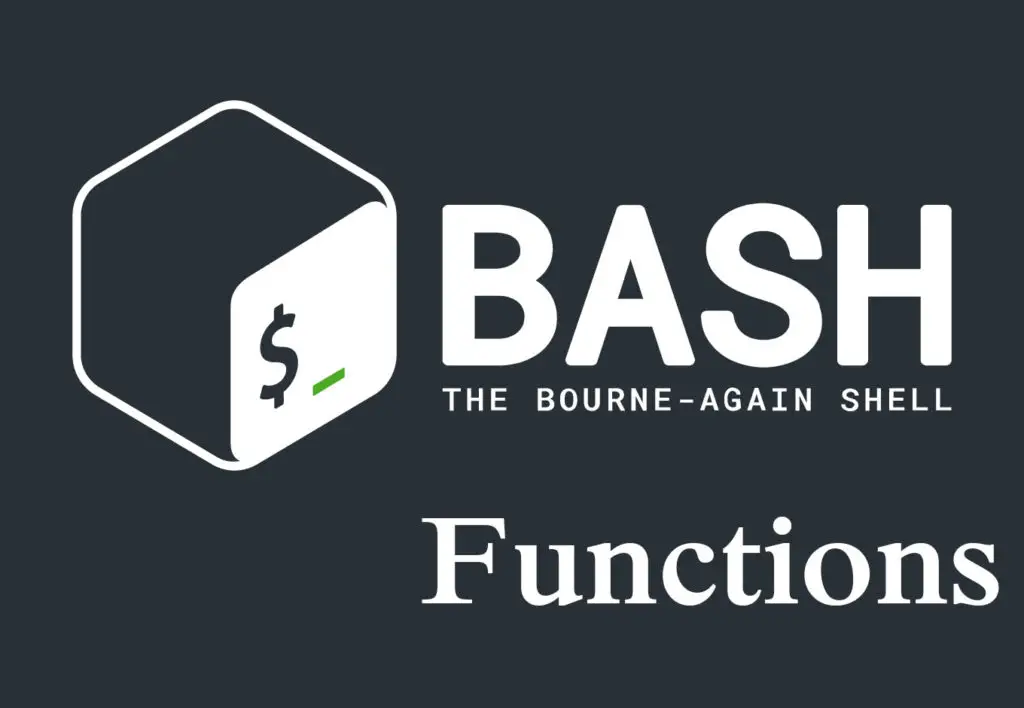
A BASH Function is a set of commands used to perform a specific task within a script. This function can be reused anywhere in your script to perform that particular task several times. The function saves our time by the need of writing the same code over and over again with write it once and call it every time.
In this tutorial, we will see the basics of bash functions and learn how to use them in shell scripts.
Simple Function
Creating a function is easy and written in two formats.
Syntax 1
This format begins with the function name followed by parentheses.
function_name () { <commands>; } function_name
Syntax 2
This format begins with the syntax function followed by the function name.
function function_name () { <commands>; } function_name
Notes
- Both syntaxes should just work fine, and there is no functionality difference.
- Semicolon is optional
- To execute a function, you must invoke a function using the function name (function_name) whenever required in the shell script
Create a shell script hello_world.sh
with the following code.
#!/bin/bash #Basic Function #Function Name func_helloworld () { #commands echo "Hello World"; } #Invoking Function func_helloworld #Reusing Function func_helloworld func_helloworld
Let’s execute this script with the bash shell. Running the above function will produce the following output.
[root@server ~]# chmod +x hello_world.sh [root@server ~]# ./hello_world.sh Hello World Hello World Hello World [root@server ~]#
Passing Arguments to Bash Functions
We may not always want a simple static function. Sometimes, we need the function to process the input for us just like we pass argument(s) to bash commands in shell prompt.
In function, we supply the arguments after we call a function in a script.
The first parameter is referred to in the function as $1, the second as $2, etc.
#!/bin/bash #Passing Arguments to a function func_welcome () { echo Hello $1 Welcome to $2 } #Calling a Function with Parameters func_welcome Raj ITzGeek
Let’s execute this script with the bash shell. Running the above function will produce the following output.
[root@server ~]# chmod +x arguments.sh [root@server ~]# ./arguments.sh Hello Raj Welcome to ITzGeek [root@server ~]#
Variable Scope
There are two types of variable
Global Variable
Global variables are variables that can be called from anywhere in the script regardless of where it is defined, inside or outside a function. In Bash, all variables are by default global variable.
var_name=<var_value>
Local Variable
Local variables are are defined within the function {} with the keyword local. Local variables scope is limited and works only within that function.
local var_name=<var_value>
Let’s create a script with global and local variables and see how they work within and outside the function.
#!/bin/bash #Variable Scope #Global Variable var1='X' var2='Y' func_varscope () { #Local Variable local var1='Z' #Gloabl Variable var2='A' echo "Inside a Function: var1 is $var1 and var2 is $var2" } echo "Before Calling a Function: var1 is $var1 and var2 is $var2" func_varscope echo "After Calling a Function: var1 is $var1 and var2 is $var2"
The above script starts with defining two global variables var1 and var2 and then inside the function a local variable var1 and another global variable with the same name var2.
Let’s execute this script with the bash shell.
Before Calling a Function: var1 is X and var2 is Y Inside a Function: var1 is Z and var2 is A After Calling a Function: var1 is X and var2 is A
If you see the above output,
Setting a local variable inside the function with the same as an existing global variable modifies the global variable value and only to the function. Outside the function, the variable value is equal to the global variable.
Global variable within the function can modify the global variable outside the function.
Return Values
While other programming languages return the value of functions, Bash functions don’t return value when called. However, Bash functions allow us to set a return status.
We can use the keyword return to specify the return status.
#!/bin/bash func_returnvalues () { echo "some results" return 55 } func_returnvalues echo $?
Let’s execute this script with the bash shell.
some results 55
If you want to return the actual value of functions, we need to assign the result of the function to a variable.
Global Variable
#!/bin/bash func_returnvalues () { func_result="some result" } func_returnvalues echo $func_result
Let’s execute this script with the bash shell.
some result
Local Variable
#!/bin/bash func_returnvalues () { local func_result="some result" echo $func_result } func_result=$(func_returnvalues) echo $func_result
Let’s execute this script with the bash shell.
some result
Conclusion
Creating functions in a Bash script is easy. Using functions in your script would save you lots of time by reusing them whenever required. Keep your Bash function as simple as possible and break a large function into smaller ones if you found one in your scripts.